Using Let and Context to Modularize RSpec Tests
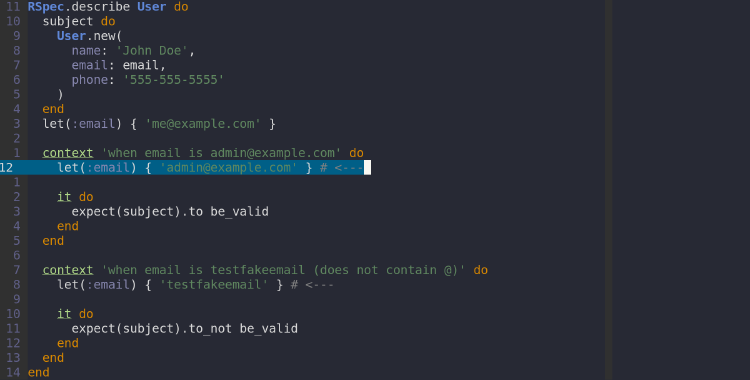
When test suites contain a lot of duplication, coupling occurs at an individual spec basis. By utilizing core RSpec functionality, developers can clean up specs in order to reduce duplication and add clarity to the focal point of the spec.
If I want to test an invalid and valid email, it’s pretty easy to test with a basic RSpec test:
RSpec.describe User do
it 'me@example.com is a valid email' do
expect(
User.new(
name: 'John Doe',
email: 'john@example.com',
phone: '555-555-5555'
)
).to be_valid
end
it 'johnatexampledotcom is not a valid email' do
expect(
User.new(
name: 'John Doe',
email: 'johnatexampledotcom',
phone: '555-555-5555'
)
).to_not be_valid
end
end
While this may work for the first few specs, this starts to get a bit tedious and duplicative.
This is not optimal because our setup code has a lot of duplication and this duplicated code.
The RSpec Let + Context Pattern
By using core Rspec functionality, we can spruce up these 2 tests like so:
RSpec.describe User do
subject do
User.new(
name: 'John Doe',
email: email,
phone: '555-555-5555'
)
end
let(:email) { 'me@example.com' }
context 'when email is admin@example.com' do
let(:email) { 'admin@example.com' }
it do
expect(subject).to be_valid
end
end
context 'when email is testfakeemail (does not contain @)' do
let(:email) { 'testfakeemail' }
it do
expect(subject).to_not be_valid
end
end
end
This provides us with:
- DRY specs
- Re-usable setup code
- Additional specs within each context
- New specs, such as “phone number validations”
- Easily interpretable differences between specs
The context / let pattern brings the most clarity into the differences between the 2 tests. This pattern can be used with in combination with Factories in order to make my tests extra clear and extra dry. Additionally, this pattern typically results in a more thoughtful test output.